Performance Considerations in building CRUD Interfaces
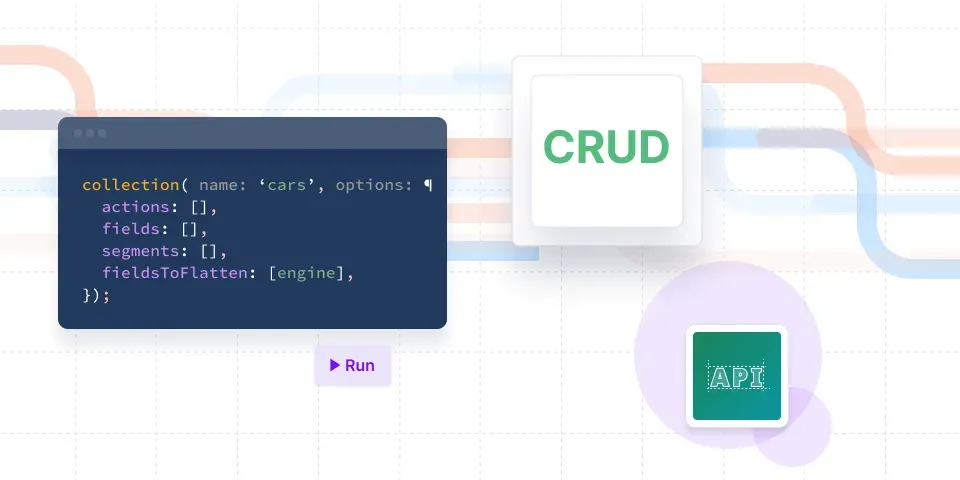
Creating a CRUD interface might seem easy, but sometimes it doesn't perform as well as we'd hope. One very common issue is poor performance, which can make using CRUD interfaces frustrating, especially with prolonged use.
In this blog post, we'll share a few aspects to remember when building CRUD interfaces to ensure they operate at their best.
Efficient Database Queries
At the heart of any CRUD interface lies its interaction with the database. Inefficient database queries can significantly impact CRUD performance, leading to slow response times and increased resource consumption. It's crucial to optimize queries, utilize proper indexing, and minimize unnecessary data retrieval to enhance performance.
Bad approach
SELECT *
FROM products
WHERE category = 'Electronics' AND price < 100;
This query retrieves all columns from the products
table where the category is 'Electronics' and the price is less than 100, potentially leading to slow performance.
Good approach
SELECT id, name, description
FROM products
WHERE category = 'Electronics' AND price < 100;
This query selects only the necessary columns from the products
table and benefits from indexing for better performance.
Caching Mechanisms
PostgreSQL employs a built-in cache, known as the Shared Buffer Cache, to efficiently store frequently accessed data in memory. This cache mechanism helps reduce the need for disk I/O operations, thereby speeding up query execution for CRUD performance. Monitoring cache usage in PostgreSQL involves examining statistics views such as pg_stat_user_tables
, which provide insights into the frequency of cache hits versus disk reads for individual database tables. By analyzing these statistics, administrators can assess the effectiveness of caching strategies and fine-tune database performance accordingly.
Implementing an application-level caching strategy can enhance PostgreSQL's built-in cache. Application-level caching involves storing frequently accessed data in memory within the application. Developers can customize caching policies and strategies to suit specific application requirements, such as setting expiration times or implementing cache invalidation mechanisms.
Example
const redis = require("redis");
const client = redis.createClient();
function fetchData(key) {
return new Promise((resolve, reject) => {
client.get(key, (err, data) => {
if (err) reject(err);
else resolve(data || fetchDataFromDatabase(key));
});
});
}
function fetchDataFromDatabase(key) {
const newData = "Data fetched from the database for key: " + key;
client.set(key, newData);
return newData;
}
fetchData("key1").then(data => console.log(data));
Optimizing Relationships
One typical example of handling relationships poorly is by making excessive use of joins in relational databases. Excessive joins involve combining data from multiple tables based on related columns, which can lead to complex and resource-intensive queries. Each join operation adds overhead in terms of processing time and disk I/O, especially if the joined tables are large or if indexes are not properly utilized. As a result, queries with excessive joins may experience slow execution times and strain database resources, leading to degraded performance and potential scalability issues.
Another example of CRUD performance issues related to relationships is seen in the context of dropdown menus. For instance, loading all related records in a dropdown, such as all orders associated with a customer, can cause significant performance problems, particularly with large datasets. Instead, utilizing techniques like lazy loading and filtering can help mitigate these issues by fetching and displaying only the necessary data as required, thereby improving performance and user experience.
Bad approach: Loading all orders into a dropdown
populateDropdown(orders);
Good approach: using a Typeahead field with a filtered dropdown and server-side data fetch
typeaheadField.addEventListener('input', function() {
fetch('/api/orders?q=' + query)
.then(response => response.json())
.then(orders => populateDropdown(orders));
});
Addressing Overfetching and Underfetching
Overfetching and underfetching data are common pitfalls that can hinder performance in a CRUD interface. Fetching more data than necessary leads to increased network bandwidth and processing overhead, while fetching too little data may necessitate additional round trips to the database. Striking the right balance and optimizing data retrieval mechanisms are crucial for optimal CRUD performance.
Leveraging Asynchronous Operations
Utilizing asynchronous programming techniques can significantly enhance the scalability and responsiveness of a CRUD interface. By executing operations concurrently and non-blocking, asynchronous operations minimize idle time and maximize resource utilization. Incorporating asynchronous paradigms into your application architecture can lead to substantial performance improvements.
Bad approach
This code executes fetch requests sequentially. It waits for the response of the first fetch request to complete before initiating the second one, leading to slower overall performance.
const response1 = await fetch('api/customers/1/orders');
const response2 = await fetch('api/customers/2/orders');
const orders1 = await response1.json();
const orders2 = await response2.json();
processData(orders1, orders2);
Good approach
In this good approach, both fetch
calls are executed simultaneously using Promise.all
, which allows for parallel fetching of data. This can result in better performance compared to fetching data sequentially. Once both responses are received, their JSON data is processed asynchronously using await
.
const [response1, response2] = await Promise.all([
fetch('api/customers/1/orders'),
fetch('api/customers/2/orders')
]);
const orders1 = await response1.json();
const orders2 = await response2.json();
processData(orders1, orders2);
Mitigating Network Latency
Reducing network latency is crucial for enhancing the performance of CRUD interfaces, especially in scenarios involving remote servers or APIs. Employing efficient data transfer protocols, such as JSON API or GraphQL, allows for embedding large datasets and their relationships in a single payload, minimizing the number of round trips between the client and server. By consolidating data retrieval into a single efficient request, developers can mitigate latency and create more responsive and scalable web applications.
Bad approach
const booksResponse = await fetch('api/books');
const booksData = await booksResponse.json();
const authorsResponse = await fetch('api/authors');
const authorsData = await authorsResponse.json();
Good approach
const response = await fetch('api/books?include=author');
const data = await response.json();
/* Example of response data
{
"data": [
{
"type": "book",
"id": "1",
"attributes": {
"title": "Book 1"
},
"relationships": {
"author": {
"data": { "type": "author", "id": "1" }
}
}
},
{
"type": "book",
"id": "2",
"attributes": {
"title": "Book 2"
},
"relationships": {
"author": {
"data": { "type": "author", "id": "2" }
}
}
}
],
"included": [
{
"type": "author",
"id": "1",
"attributes": {
"name": "Author 1"
}
},
{
"type": "author",
"id": "2",
"attributes": {
"name": "Author 2"
}
}
]
}
*/
Conclusion
Building a high-performance CRUD interface requires careful consideration of various factors, from database optimization to code structure and network communication. By addressing inefficiencies, implementing best practices, and continuously optimizing performance, developers can create CRUD interfaces that deliver exceptional user experiences and operate seamlessly under demanding conditions.
Remembering these key aspects and prioritizing CRUD performance optimization throughout the development lifecycle is essential for building robust and efficient interfaces.