User Authorization: A Guide for Developers
This guide provides insights into authentication vs authorization, role-based access control (RBAC), OAuth 2.0, JSON Web Tokens (JWT), and best practices for implementing user authorization in an application.
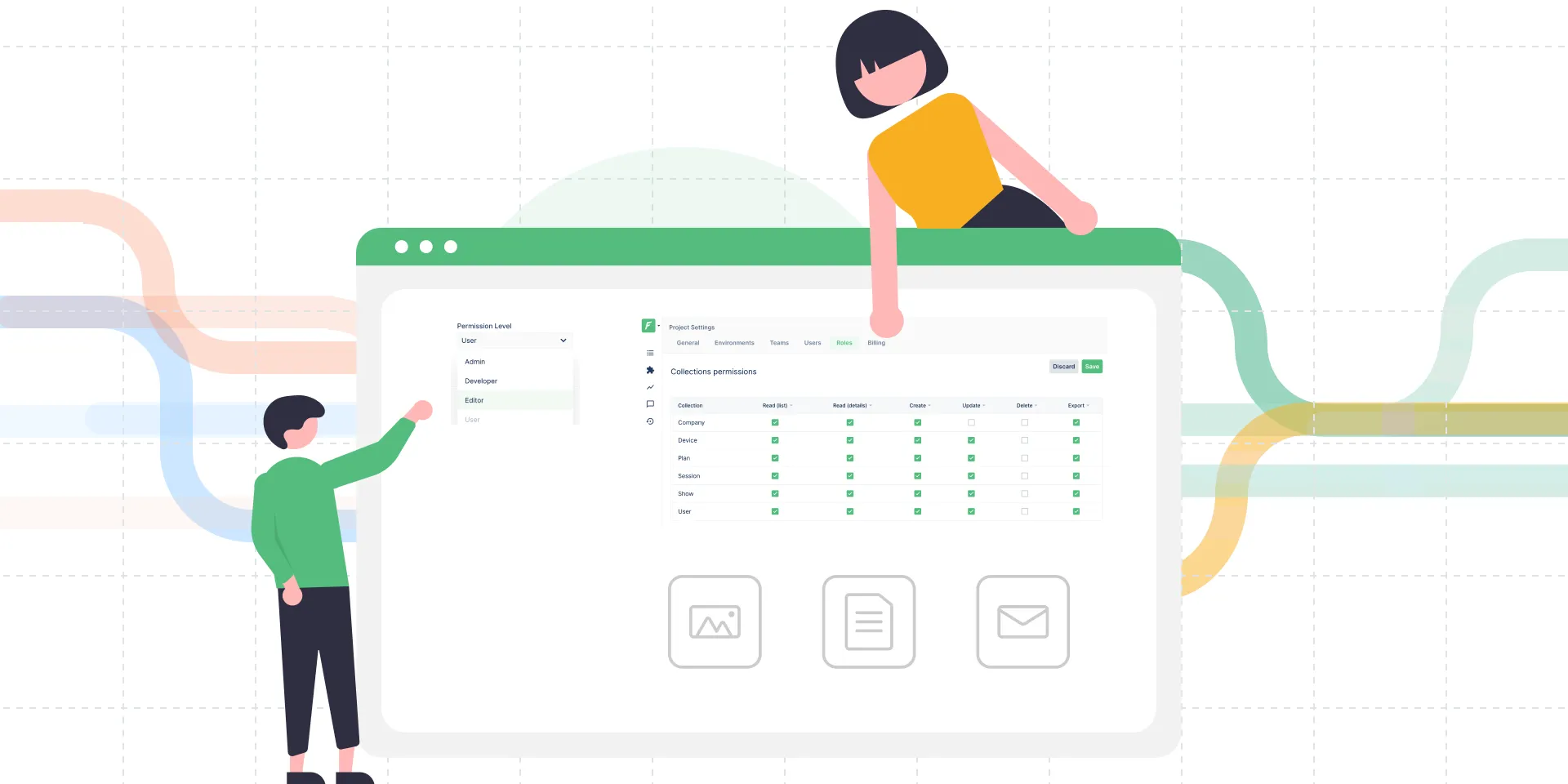
User authorization is a crucial aspect of application development, ensuring that only authorized users have access to specific resources and functionalities. It is the process of granting permissions to users based on their identity and role within the system. By implementing user authorization, developers can enforce security measures and protect sensitive data.
Developers need to understand the different user authorization methods and choose the most suitable approach for their application. This guide will provide insights into authentication vs authorization, role-based access control (RBAC), OAuth 2.0, JSON Web Tokens (JWT), and best practices for implementing user authorization in an application.
What is User Authorization?
User authorization is a fundamental concept in application development that involves granting or denying access to specific resources or functionalities based on a user's identity and role within the system. It is a crucial component of ensuring the security and integrity of an application.
When a user attempts to access certain features or data within an application, the authorization process verifies their identity and checks if they have the necessary permissions to perform the requested action. This process involves evaluating the user's credentials, roles, and permissions against predefined rules and policies.
User authorization is closely related to authentication, which is the process of verifying a user's identity. However, while authentication focuses on confirming that a user is who they claim to be, authorization determines what actions that user is allowed to perform within the application.
There are various methods and techniques for implementing user authorization, including role-based access control (RBAC), where access is granted based on a user's assigned role or group membership. Another common approach is OAuth 2.0, which allows users to grant limited access to their resources to third-party applications. JSON Web Tokens (JWT) are also widely used for securely transmitting authorization information between parties.
Overall, user authorization plays a critical role in maintaining the security and privacy of an application. By properly implementing user authorization, developers can ensure that only authorized individuals have access to sensitive data and functionalities, protecting the application and its users from unauthorized access and potential security breaches.
Authentication vs Authorization
Authentication and authorization are two distinct but closely related concepts in the field of application security. While they are often used together, it's important to understand the differences between them.
Authentication is the process of verifying the identity of a user or entity. It ensures that the user is who they claim to be by validating their credentials, such as a username and password, or using other authentication methods like biometrics or two-factor authentication. The main goal of authentication is to establish trust and confirm the user's identity.
Authorization, on the other hand, is the process of granting or denying access to specific resources or functionalities based on the authenticated user's identity and permissions. It determines what actions the user is allowed to perform within the system. Authorization is about controlling access and ensuring that users only have access to the resources they are authorized to use.
In simpler terms, authentication is about verifying who you are, while authorization is about determining what you can do. Authentication establishes trust, and authorization enforces restrictions and permissions.
Both authentication and authorization are critical for application security. Implementing strong authentication mechanisms ensures that only legitimate users can access the system, while proper authorization mechanisms protect sensitive data and functionalities from unauthorized access.
It's important for developers to understand the differences between authentication and authorization and implement them correctly in their applications to ensure the security and integrity of their systems.
Common User Authorization Methods
There are several common user authorization methods used in application development to control access to resources and functionalities:
- Role-Based Access Control (RBAC): This method assigns roles to users and grants permissions based on those roles. It allows for easy management of user access by grouping users with similar responsibilities.
- OAuth 2.0: OAuth 2.0 is an authorization framework that allows users to grant limited access to their resources to third-party applications without sharing their credentials. It is commonly used for API authorization.
- JSON Web Tokens (JWT): JWT is a compact and self-contained method for transmitting authorization information between parties. It is commonly used for stateless authentication and authorization in web applications.
These methods provide developers with flexible and scalable ways to implement user authorization in their applications, depending on their specific requirements and use cases.
Role-Based Access Control (RBAC)
Role-Based Access Control (RBAC) is a widely used user authorization method that provides a flexible and scalable approach to controlling access to resources and functionalities within an application. It is based on the concept of assigning roles to users and granting permissions based on those roles.
In RBAC, users are assigned one or more roles that define their responsibilities and access rights. Each role is associated with a set of permissions that determine what actions the user can perform within the system. This allows for a more granular and organized approach to managing user access.
RBAC offers several benefits for developers and system administrators. It simplifies user management by grouping users with similar responsibilities into roles, reducing the complexity of assigning and revoking individual permissions. It also enhances security by ensuring that users only have access to the resources and functionalities necessary for their roles.
RBAC can be implemented at different levels, including the application level, where roles and permissions are defined within the application itself, or at the infrastructure level, where RBAC frameworks and tools are used to manage user access across multiple applications and systems.
Implementing RBAC requires careful planning and design. Developers need to define roles and associated permissions based on the specific requirements of their application. They also need to consider factors such as user hierarchy, role inheritance, and dynamic role assignment to ensure an effective and efficient RBAC system.
Overall, Role-Based Access Control (RBAC) is a powerful user authorization method that provides a structured and scalable approach to controlling access in applications. By implementing RBAC, developers can enhance security, simplify user management, and ensure that users have appropriate access to resources and functionalities based on their roles. Read more about Access Control Guidelines.
OAuth 2.0
OAuth 2.0 is an authorization framework that allows users to grant limited access to their resources to third-party applications without sharing their credentials. It provides a secure and standardized way for applications to obtain authorization from users to access their protected resources.
Permission Management: With OAuth 2.0, users can grant permissions to third-party applications by authenticating with the authorization server and providing consent. The third-party application then receives an access token, which it can use to access the user's resources on their behalf. This allows for secure and controlled access to user data without exposing sensitive information.
OAuth 2.0 is widely used for API authorization and is supported by many popular platforms and services, including social media platforms and cloud service providers. It offers several advantages, such as improved security by eliminating the need for applications to store user credentials and the ability for users to revoke access at any time.
Developers can implement OAuth 2.0 in their applications by integrating an OAuth 2.0 client library or using a framework that provides OAuth 2.0 support. They need to register their application with the authorization server, configure the required scopes and permissions, and handle the OAuth 2.0 flow to obtain and refresh access tokens.
OAuth 2.0 provides a flexible and standardized approach to user authorization for third-party applications. By leveraging OAuth 2.0, developers can enhance security, improve user experience, and enable seamless integration with other applications and services.
JSON Web Tokens (JWT)
JSON Web Tokens (JWT) is a widely used method for securely transmitting authorization information between parties. It is a compact and self-contained format that can be used to represent claims between two entities, typically a user and a server.
A JWT consists of three parts: a header, a payload, and a signature. The header contains information about the type of token and the signing algorithm used. The payload contains the claims, which are statements about the user and additional data. The signature is used to verify the authenticity of the token.
JWTs are commonly used for stateless authentication and authorization in web applications. When a user logs in, the server generates a JWT and sends it back to the client. The client then includes the JWT in subsequent requests to the server, which can verify the authenticity of the token and authorize the requested actions based on the claims within the JWT.
One of the key advantages of JWTs is that they can be easily verified without the need for a centralized authentication server. This makes JWTs a scalable solution for distributed systems and microservices architectures.
Developers can implement JWT authentication and authorization by integrating JWT libraries or frameworks into their applications. They need to securely generate and sign JWTs, handle token validation and expiration, and define the appropriate claims for their application's authorization requirements.
JSON Web Tokens provide a secure and efficient way to transmit authorization information between parties. By leveraging JWTs, developers can implement robust authentication and authorization mechanisms in their applications, ensuring the integrity and security of user data and resources.
Implementing User Authorization in Your Application
Implementing user authorization in your application is crucial for ensuring the security and integrity of your system. It involves controlling access to resources and functionalities based on user roles, permissions, and authentication. By following best practices and using appropriate methods such as Role-Based Access Control (RBAC), OAuth 2.0, or JSON Web Tokens (JWT), you can effectively manage user access and protect sensitive data. Consider designing an authorization model, integrating user authentication, and regularly reviewing and updating user permissions. By properly implementing user authorization, you can enhance the security and user experience of your application.
Designing an Authorization Model
Designing an authorization model is a crucial step in implementing user authorization in your application. It involves defining the structure and rules that determine how users are granted access to resources and functionalities.
When designing an authorization model, consider the following:
- User Roles: Identify the different roles that users can have within your application. Roles define the level of access and permissions for each user.
- Permissions: Determine the specific actions or operations that users can perform within your application. Assign permissions to roles based on the required level of access.
- Hierarchy: Establish a hierarchy among roles if necessary. Some roles may have higher user privileges and can perform actions that lower-level roles cannot.
- Grouping and Inheritance: Group roles with similar access requirements together. Consider role inheritance, where lower-level roles inherit permissions from higher-level roles.
- Dynamic Authorization: Plan for scenarios where authorization rules may change dynamically. Consider implementing a flexible authorization model that allows for easy modification of user access based on changing requirements.
By carefully designing your authorization model, you can ensure that access to resources and functionalities is granted in a structured and controlled manner. This helps maintain the security and integrity of your application while providing a seamless and efficient user experience.
Integrating User Authentication
Integrating user authentication is a critical part of implementing user authorization in your application. User authentication verifies the identity of users and ensures that only authenticated users can access protected resources and functionalities.
When integrating user authentication, consider the following:
- Authentication Methods: Choose the appropriate authentication methods based on your application's requirements. Common methods include username/password authentication, social media login, or single sign-on (SSO) with external identity providers.
- Secure Storage: Safely store user credentials to prevent unauthorized access. Use secure hashing algorithms and encryption techniques to protect passwords and sensitive user information.
- Authentication Frameworks: Leverage authentication frameworks and libraries to simplify the implementation process. Popular frameworks such as OAuth, OpenID Connect, or IdentityServer provide pre-built components and security features.
- Multi-Factor Authentication (MFA): Consider implementing MFA to add an extra layer of security. This can include methods such as SMS verification, email verification, or authenticator apps.
- Session Management: Manage user sessions securely to maintain authentication state and prevent session hijacking or session fixation attacks.
By integrating user authentication effectively, you can ensure that only authenticated users can access your application's protected resources. This enhances the security of your application and provides a seamless and trusted user experience.
Implementing Role-Based Access Control
Implementing Role-Based Access Control (RBAC) is a powerful approach to user authorization in your application. RBAC allows you to define roles and assign specific permissions to each role, granting users access based on their assigned roles.
When implementing RBAC, consider the following steps:
- Identify Roles: Determine the different roles that exist within your application. Roles can represent different job positions, user types, or levels of access.
- Define Permissions: Identify the specific actions or operations that users can perform within your application. Map these permissions to each role, ensuring that roles have appropriate and necessary access.
- Assign Roles: Assign roles to users based on their responsibilities or access requirements. Users can have multiple roles if needed.
- Role Inheritance: Consider implementing role inheritance, where lower-level roles inherit permissions from higher-level roles. This simplifies role management and allows for a hierarchical role structure.
- Manage Role Changes: Implement mechanisms for adding, modifying, or revoking roles and permissions as user requirements change over time.
By implementing RBAC, you can ensure that users have the appropriate level of access to resources and functionalities based on their assigned roles. This enhances security, simplifies user management, and provides a scalable solution for authorization in your application.
Best Practices for User Authorization
Implementing user authorization in your application requires following best practices to ensure the security and effectiveness of your authorization system. Consider the following best practices:
- Keep Authorization Logic Separate: Separate authorization logic from other application code to improve maintainability and reduce security vulnerabilities.
- Use Strong Password Policies: Enforce strong password policies, such as minimum length, complexity requirements, and regular password expiration.
- Regularly Review and Update User Permissions: Conduct regular reviews of user permissions to ensure they align with their roles and responsibilities. Remove or update permissions as needed.
By following these best practices, you can enhance the security and efficiency of your user authorization system, ensuring that users have appropriate access to resources and functionalities while protecting sensitive data from unauthorized access.
Keep Authorization Logic Separate
Keeping authorization logic separate is a crucial best practice in user authorization. By separating authorization logic from other application code, you can improve the maintainability, scalability, and security of your application.
Separating authorization logic involves isolating the code that handles user authentication, permission checks, and access control into its own module or component. This allows you to manage authorization rules independently and make changes without affecting the rest of the application.
Benefits of keeping authorization logic separate include:
- Maintainability: It becomes easier to understand and modify authorization logic, as it is isolated from other application functionalities. This improves code readability and facilitates future updates or enhancements.
- Security: Separating authorization logic helps minimize the risk of security vulnerabilities. It allows for focused testing and auditing of the authorization module, reducing the likelihood of unintended access or privilege escalation.
- Scalability: With separate authorization logic, you can easily integrate new authentication mechanisms, implement additional authorization rules, or handle complex access control scenarios.
By keeping authorization logic separate, you can ensure that your application's authorization system remains robust, maintainable, and adaptable to future changes. It also helps enforce the principle of least privilege, ensuring that users only have access to the resources and functionalities they need.
Use Strong Password Policies
Using strong password policies is a vital best practice in user authorization to enhance the security of your application. Strong passwords help protect user accounts from unauthorized access and minimize the risk of security breaches.
When implementing strong password policies, consider the following:
- Minimum Complexity: Set a minimum length and require a combination of uppercase and lowercase letters, numbers, and special characters.
- Regular Expiration: Enforce password expiration to prompt users to update their passwords periodically. This helps prevent the use of compromised passwords over an extended period.
- Password History: Keep track of users' previous passwords to prevent them from reusing the same or similar passwords, which can be vulnerable to attacks.
- Account Lockouts: Implement account lockouts after a certain number of failed login attempts to prevent brute-force attacks.
By implementing strong password policies, you can significantly reduce the risk of unauthorized access to user accounts and protect sensitive data. However, it's essential to balance password complexity requirements with usability to ensure a positive user experience.
Additionally, educating users about the importance of creating strong passwords and regularly updating them can further enhance the security of your application.
Regularly Review and Update User Permissions
Regularly reviewing and updating user permissions is a critical best practice in user authorization. It ensures that users have the appropriate level of access to resources and functionalities based on their roles and responsibilities within the application.
When implementing this best practice, consider the following:
- Periodic Reviews: Conduct regular reviews of user permissions to ensure they align with current organizational requirements and user responsibilities. This can be done on a quarterly, semi-annual, or annual basis.
- Role Changes: Identify any changes in user roles or responsibilities and adjust their permissions accordingly. Remove permissions that are no longer necessary or assign additional permissions as needed.
- Revocation of Access: Promptly revoke access for users who no longer require it, such as employees who have left the organization or contractors whose projects have ended.
- Documentation: Maintain clear and up-to-date documentation of user roles, permissions, and the process for requesting and granting access. This helps ensure consistency and accountability.
Regularly reviewing and updating user permissions helps maintain the security and integrity of your application. It minimizes the risk of unauthorized access and ensures that users only have access to the resources they need to perform their job responsibilities.
By following this best practice, you can effectively manage user permissions, reduce potential security vulnerabilities, and maintain a well-controlled user authorization system.